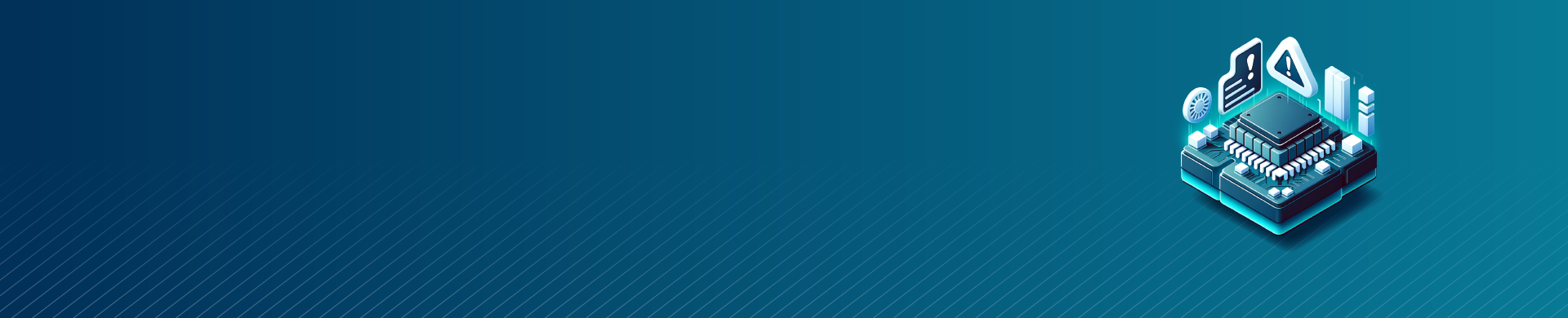
Buffer Overflow Attacks: A Dangerous Digital Threat
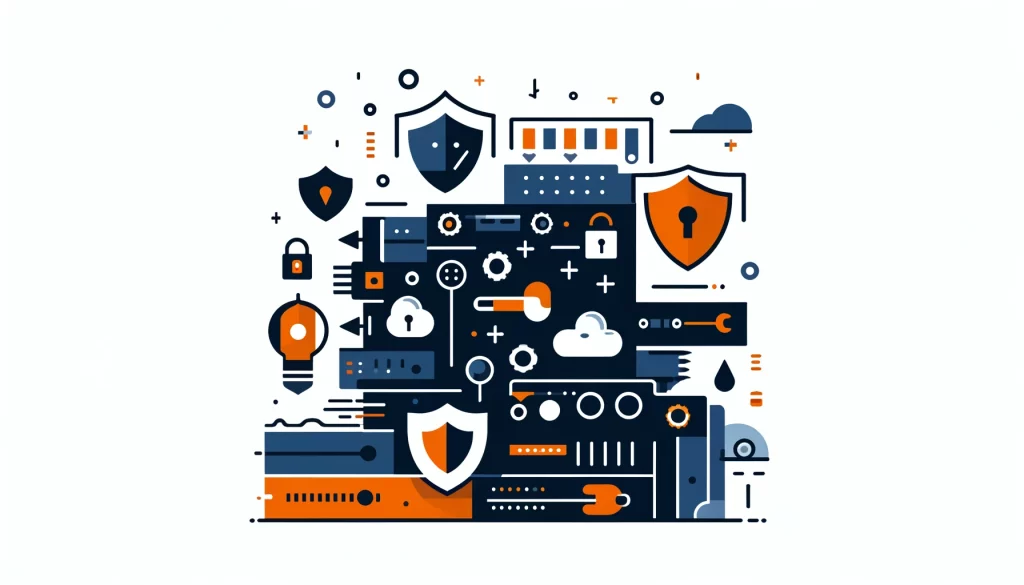
Buffer overflow attacks pose a significant threat to software security. These attacks occur when malicious individuals exploit buffer overflow vulnerabilities. This can lead to program crashes, data corruption, theft of sensitive information, and unauthorized system access. This article explains buffer overflows, attackers’ use of them, and methods to prevent them in software development.
The Basics of Buffer Overflows
A buffer, in the context of computer science, is a region of memory allocated to temporarily store data. Programs utilize buffers to hold various types of data, such as user input, file contents, or strings.
Each buffer has a set size. Issues occur when a program tries to store more data than the buffer can hold. In such cases, the excess data overflows the buffer, spilling into adjacent memory locations. This unintended behavior can lead to program crashes, data corruption, and security vulnerabilities.
Consider the following example in the C programming language:
char buffer[8]; scanf("%s", buffer);
This code snippet allocates an 8-byte buffer to store a string obtained from user input. If the user types more than 8 characters, it will overwrite nearby memory.
Exploiting Buffer Overflow Vulnerabilities
Attackers exploit buffer overflow vulnerabilities by strategically overwriting portions of memory that contain executable code, replacing it with malicious code. The attacker first identifies a program with a known buffer overflow vulnerability. The attacker purposely sends too much data to overwhelm the system and cause memory corruption.
The attacker wants to replace the buffer with malicious code. This code can do harmful actions, such as opening a shell or running commands without permission. The attack is successful when the attacker changes a function return address or exception handler. When the user executes the altered code, it runs the malicious code.
To illustrate this concept, consider a server program that receives usernames from clients. If the username storage space is 128 bytes, a hacker could send a harmful username in a certain format.
"A" * 132 + malicious_code + fake_return_address
The series of “A” characters overflows the buffer, enabling the malicious code to write into memory. The fake return address ensures that the malicious code executes when the overwritten function returns.
Real Examples of Buffer Overflow
Every buffer overflow attack boils down to the same strategy:
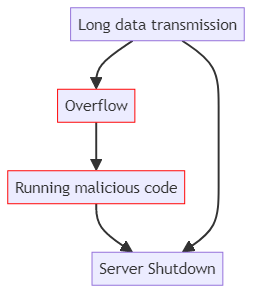
Here are some real-life examples of overflow vulnerabilities found in popular DBMS over the years.
MySQL COM_FIELD_LIST
Up until 2012 MySQL and MariaDB were prone to COM_FIELD_LIST vulnerability. The basics of the vulnerability were as follows:
Perpetrator would make a query to the MySQL Server with a ‘FROM’ clause, specifying a very long name of a table to cause buffer overflow.
SELECT * FROM 'very_long_name_of_a_table'.
The exact length was not specified, but 5000 bytes was enough to cause an overflow. This would cause the server shutdown, but in the moment between the overflow and the shutdown the server becomes vulnerable to attacks. This is where perpetrators would put malicious code in hexadecimal byte form.
SELECT * FROM 'very_long_name'.\x31\xc0\x50\x68\x2f\x2f\x73'
The code had to perform bash commands, e.g. ‘rm -rf /bin/’.
For a higher chance of success, perpetrators would add a No Operation segment to the code, in other words code that doesn’t perform anything.
A full query would look like this:
SELECT * FROM 'AAAAAAAAAAA..' causing buffer overflow '\x0\x0\x0\x0\x0' - No Operation slide '\xe3\x50\x53\x89\xe1\xb0\x0b' - Malicious code.
The vulnerability was addressed in MySQL versions 5.5.23, 5.1.63, and 5.6.6, as well as MariaDB versions 5.5.23, 5.3.12, 5.2.14, and 5.1.63. Updating to these versions or later patched the vulnerability and protected the databases from this specific attack.
PostgreSQL 9.3 RESETXLOG Buffer Overflow
In similar manner in 2013 it was discovered and quickly fixed that PostgreSQL’s pg_resetxlog() function was prone to these attacks.
postgres=# SELECT pg_resetxlog('A' * 1000 + *malicious code*);
SQL Server Slammer Worm
In 2003 SQL Server Slammer Worm attack was discovered. It targeted SQL Server Resolution server, which accepts UDP packets on port 1434. When a specially crafted packet was sent to this port, it overflowed the buffer and allowed the worm to execute arbitrary code. This code caused the server to send out more copies of the worm, leading to rapid spread and massive network congestion. An example would be like this:
payload = create_malicious_packet() sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) sock.sendto('A' * 1000, *malicious code*, (target_ip, 1434)) sock.close()
Oracle 9i XDB HTTP Buffer Overflow
In 2003 an overflow vulnerability was discovered in Oracle Server. Attackers could send a very long username in HTTP GET request, which would overflow the buffer, and then execute malicious code.
long_username = 'A' * 1000 + *malicious code* password = 'password' credentials = f"{long_username}:{password}" encoded_credentials = base64.b64encode(credentials.encode()).decode() http_request = ( "GET / HTTP/1.1\r\n" f" Host: {target_ip}\r\n" "User-Agent: Mozilla/5.0\r\n" f" Authorization: Basic {payload}\r\n" "Accept: */*\r\n" "\r\n" ) sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) sock.connect((target_ip, target_port)) sock.sendall(http_request.encode())
This snippet showcases a python script that transmits credentials to Oracle Server that overflows it’s buffer. Consequently, credentials run malicious code.
Preventing Buffer Overflow Vulnerabilities
Developing secure software requires a proactive approach to preventing buffer overflow vulnerabilities. The following strategies are essential for mitigating the risks associated with buffer overflows:
- Input Validation: Make sure all user-supplied data is within expected limits before storing it in buffers. Validate input length, format, and content to prevent malicious or unintended data from causing buffer overflows.
- Safe String and Memory Functions: Avoid using standard library functions that are prone to buffer overflows. Instead, opt for safer alternatives, such as `strncpy` and `memcpy_s`, which include built-in safeguards against overflows.
- System-Level Protections: Enable system-level security features, such as address space layout randomization (ASLR) and data execution prevention (DEP), whenever possible. These mechanisms make it harder for attackers to predict memory layouts and execute malicious code.
- Use memory-safe languages like Rust, Go, or Java to protect against buffer overflows. These languages enforce strict memory management practices and prevent direct access to memory, reducing the risk of overflow vulnerabilities.
- Regular Software Updates: Update software libraries and dependencies promptly when discovering buffer overflow vulnerabilities and releasing patches. Staying up-to-date with the latest security patches is crucial for protecting against known vulnerabilities.
Using fuzz testing tools and SAST techniques like taint analyzing can help find buffer overflow bugs while developing software.
Real-World Impact of Buffer Overflow Attacks
Throughout the history of computing, hackers have attributed numerous high-profile security incidents to buffer overflow vulnerabilities. The Morris worm was an early internet worm. It spread by exploiting a security flaw in the Unix `finger` program in 1988.
In 2017, the WannaCry virus took advantage of a weakness in the Windows Server Message Block protocol to spread. Within a single day, WannaCry infected over 230,000 computers worldwide, causing significant financial losses and disruptions.
Conclusion
Buffer overflow attacks remain a critical concern in the realm of software security. Developers can protect their software from attacks by learning about buffer overflows and how attackers exploit them.
Important to use secure coding practices. These practices include input validation and safe memory functions. They help prevent buffer overflow vulnerabilities in systems. Adopting memory-safe programming languages and keeping software dependencies up-to-date further enhances the resilience of software systems.
Software developers can improve digital system security by preventing buffer overflows and staying aware of new threats. This protects data and ensures computer systems remain reliable.