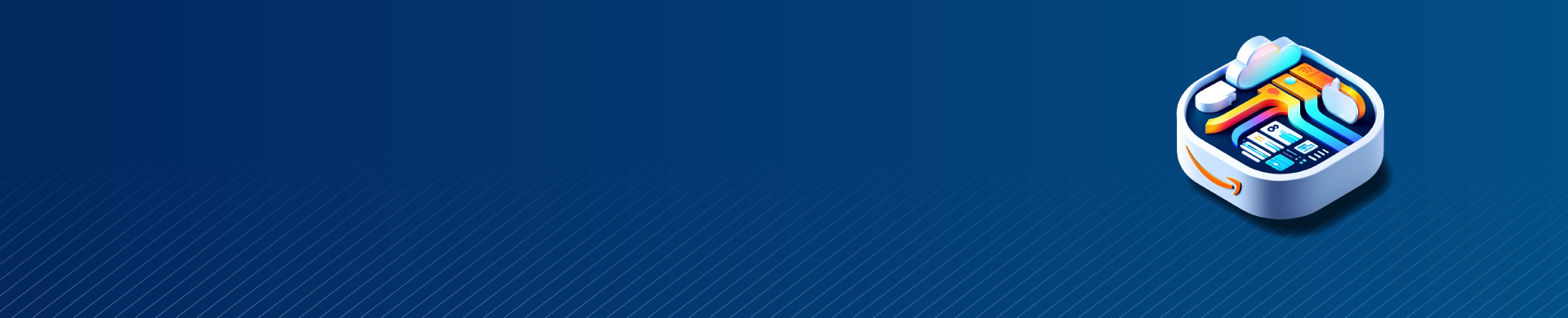
Dynamic Data Masking for Amazon Athena
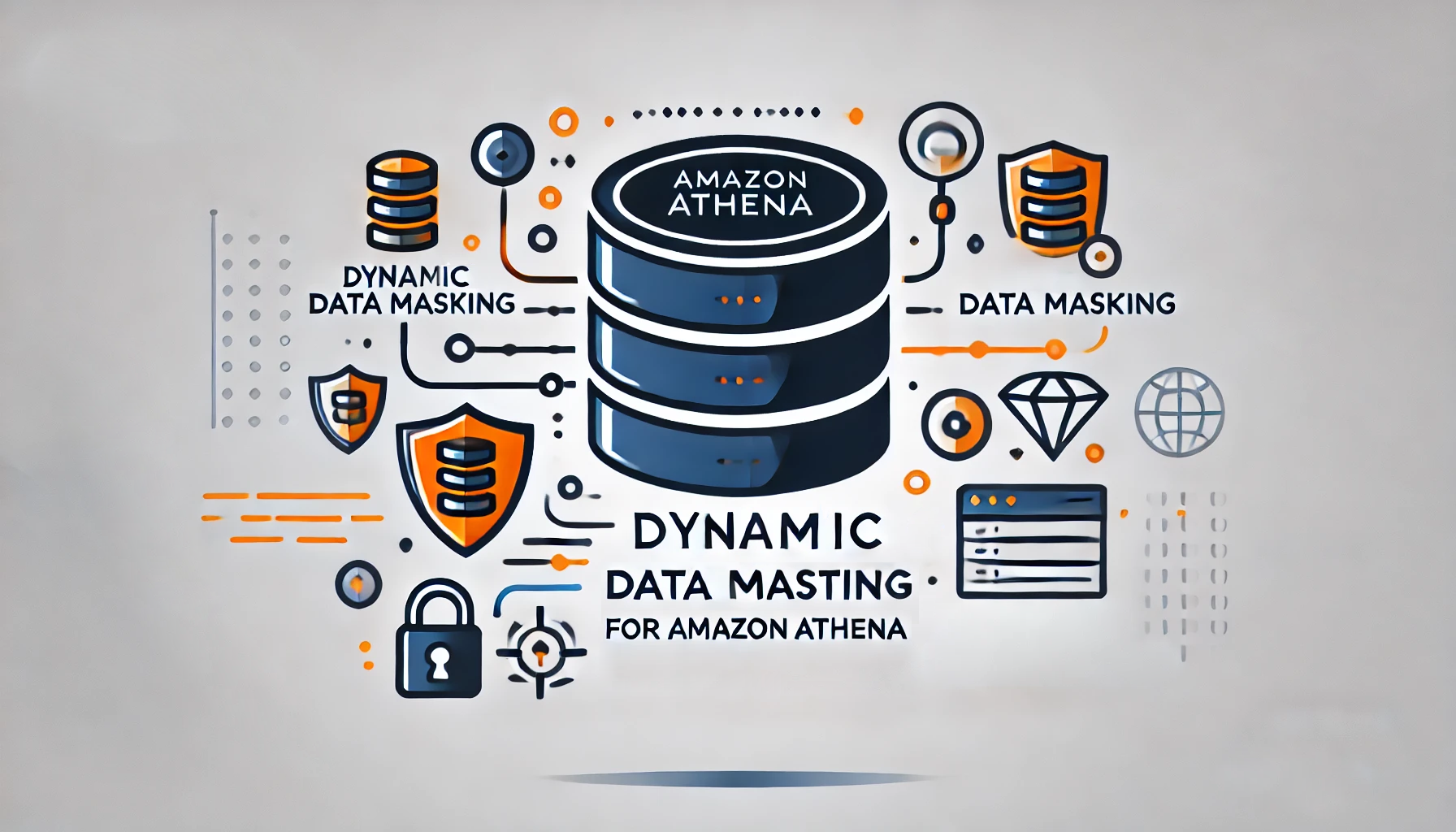
Introduction
Amazon Athena, a powerful query service, handles vast amounts of data. But how do we ensure this data remains secure? Enter dynamic data masking for Amazon Athena. This technique offers a robust solution for safeguarding sensitive data while maintaining its utility.
Large businesses are prime targets for cybercriminals due to their extensive data infrastructure and workforce. These factors often lead to more vulnerabilities compared to smaller setups. For instance, in July 2024, AT&T suffered a significant cloud infrastructure breach. This alarming trend highlights the critical need for robust data protection measures like dynamic masking.
Let’s dive into the world of dynamic data masking for Amazon Athena and explore how it can enhance your data security strategy.
Understanding Dynamic Data Masking
Dynamic data masking is a security feature that limits sensitive data exposure by masking it on-the-fly. Unlike static masking, which permanently alters data, dynamic masking preserves the original information while controlling access.
For Amazon Athena users, this means:
- Enhanced data protection
- Simplified compliance with data privacy regulations
- Flexible access control based on user roles
Now, let’s examine the various methods to implement dynamic data masking in Athena.
Native Masking with SQL Language Features
Athena supports native masking using SQL language features. This approach leverages built-in functions to mask sensitive data directly in queries.
Here’s a simple example:
SELECT id, first_name, last_name, CONCAT(SUBSTR(email, 1, 2), '****', SUBSTR(email, -4)) AS masked_email, regexp_replace(ip_address, '(\d+)\.(\d+)\.(\d+)\.(\d+)', '$1.$2.XXX.XXX') AS masked_ip FROM danielarticletable
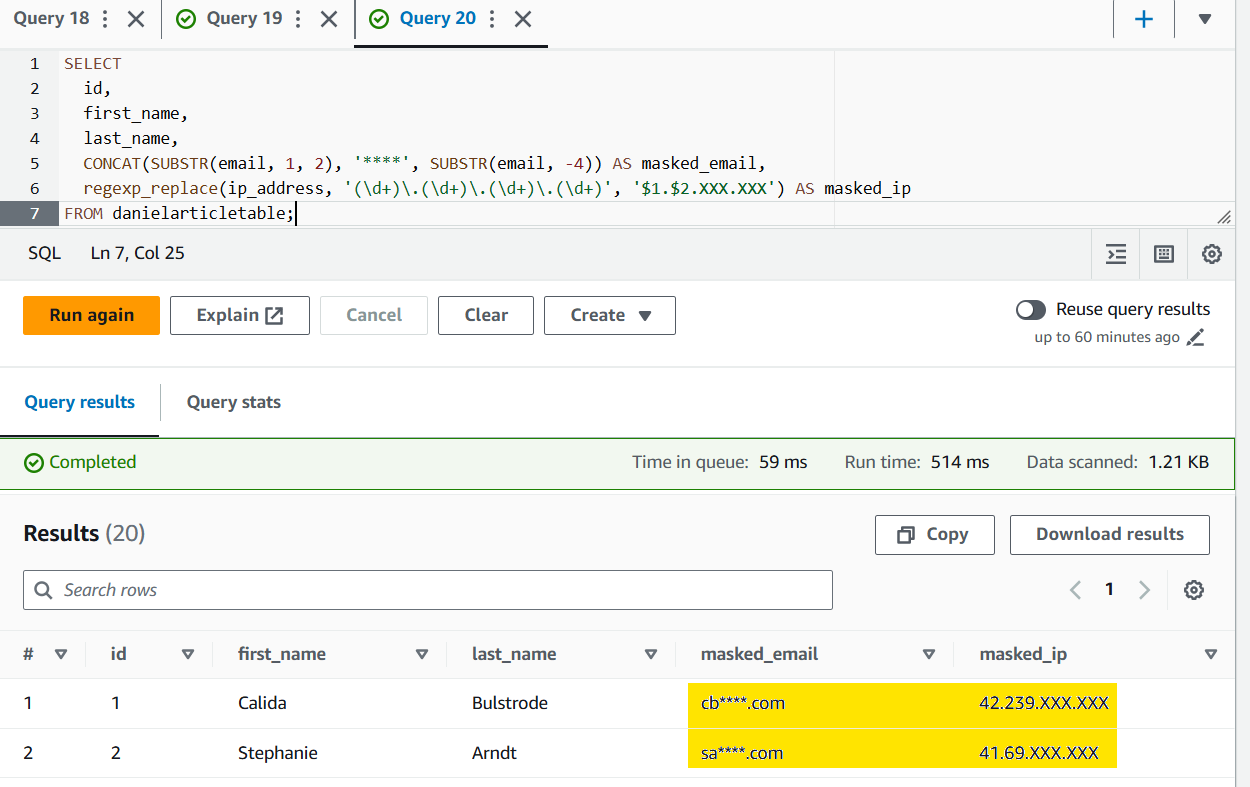
This query masks the email addresses, showing only the first two and last four characters.
Using Views for Data Masking
Views offer another native method for masking data in Athena. By creating a view with masked columns, you can control data access without modifying the underlying table.
Example:
CREATE VIEW masked_user_data AS SELECT id, first_name, last_name, CONCAT(SUBSTR(email, 1, 2), '****', SUBSTR(email, -4)) AS email, regexp_replace(ip_address, '(\d+)\.(\d+)\.(\d+)\.(\d+)', '$1.$2.XXX.XXX') AS ip_address FROM danielarticletable;
SELECT * FROM masked_user_data;
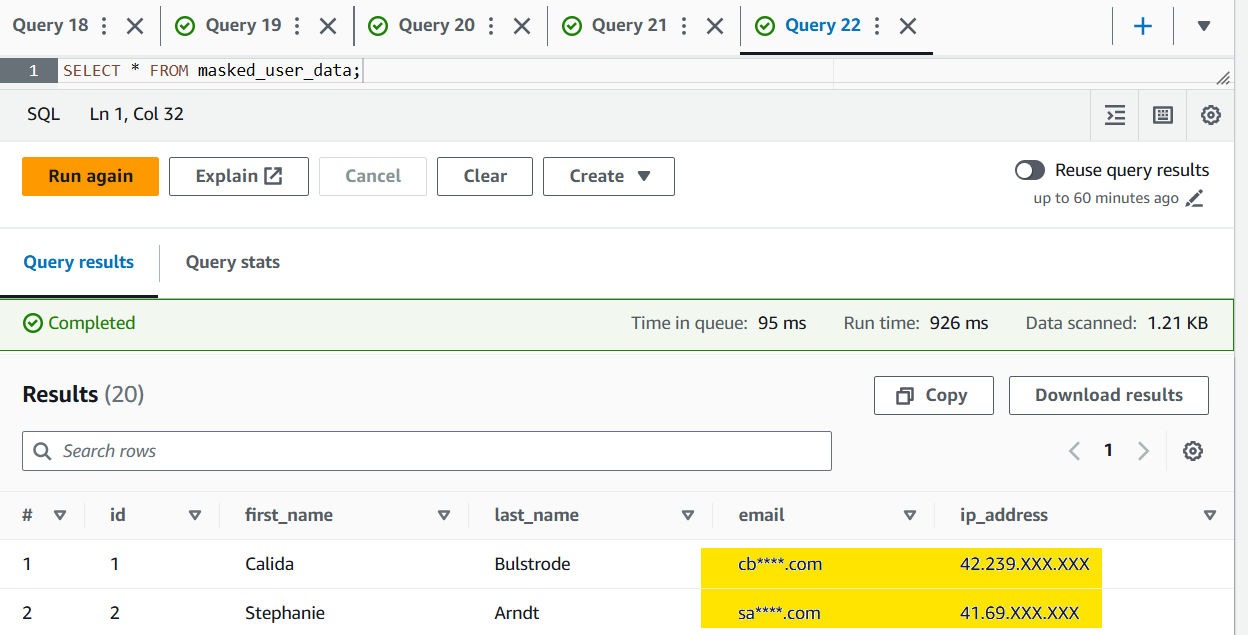
AWS CLI for Masked Data
Accessing the Athena masked view via CLI is straightforward, but requires some preparation. First, ensure you’ve configured the AWS CLI with your credentials:
aws configure
To simplify the process, we’ve compiled the necessary commands into a script. This approach streamlines interaction with Athena, as executing CLI commands individually can be cumbersome and error-prone. Make the file executable using chmod +x command.
#!/bin/bash QUERY="SELECT * FROM masked_user_data LIMIT 10" DATABASE="danielarticledatabase" S3_OUTPUT="s3://danielarticlebucket/AthenaArticleTableResults/" EXECUTION_ID=$(aws athena start-query-execution \ --query-string "$QUERY" \ --query-execution-context "Database=$DATABASE" \ --result-configuration "OutputLocation=$S3_OUTPUT" \ --output text --query 'QueryExecutionId') echo "Query execution ID: $EXECUTION_ID" # Wait for query to complete while true; do STATUS=$(aws athena get-query-execution --query-execution-id $EXECUTION_ID --output text --query 'QueryExecution.Status.State') if [ $STATUS != "RUNNING" ]; then break fi sleep 5 done if [ $STATUS = "SUCCEEDED" ]; then aws athena get-query-results --query-execution-id $EXECUTION_ID > results.json echo "Results saved to results.json" else echo "Query failed with status: $STATUS" fi
The output json file might contain data like this:
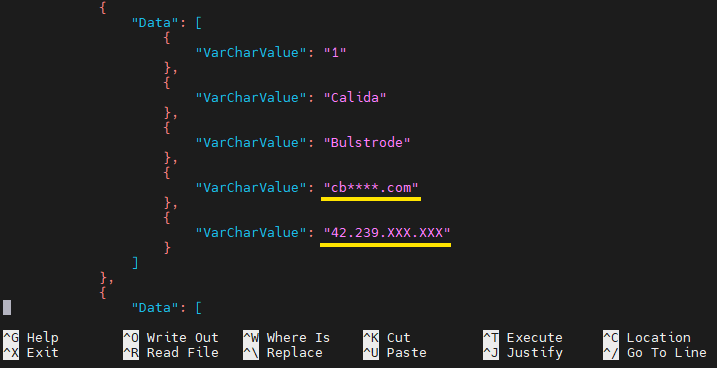
Implementing Dynamic Data Masking with Python and Boto3
For more advanced masking scenarios, Python with the Boto3 library offers greater flexibility and control. This powerful approach, which we explored in our previous article on Athena masking techniques, allows for customized and dynamic data protection solutions.
DataSunrise: Advanced Dynamic Data Masking
While Athena offers native masking capabilities, tools like DataSunrise provide more comprehensive dynamic data masking solutions. DataSunrise doesn’t support static masking for Athena, but its dynamic masking features offer powerful protection.
To use DataSunrise for dynamic masking with Athena:
- Connect DataSunrise to your Athena database
- Define masking rule in the DataSunrise interface and choose the objects to mask:
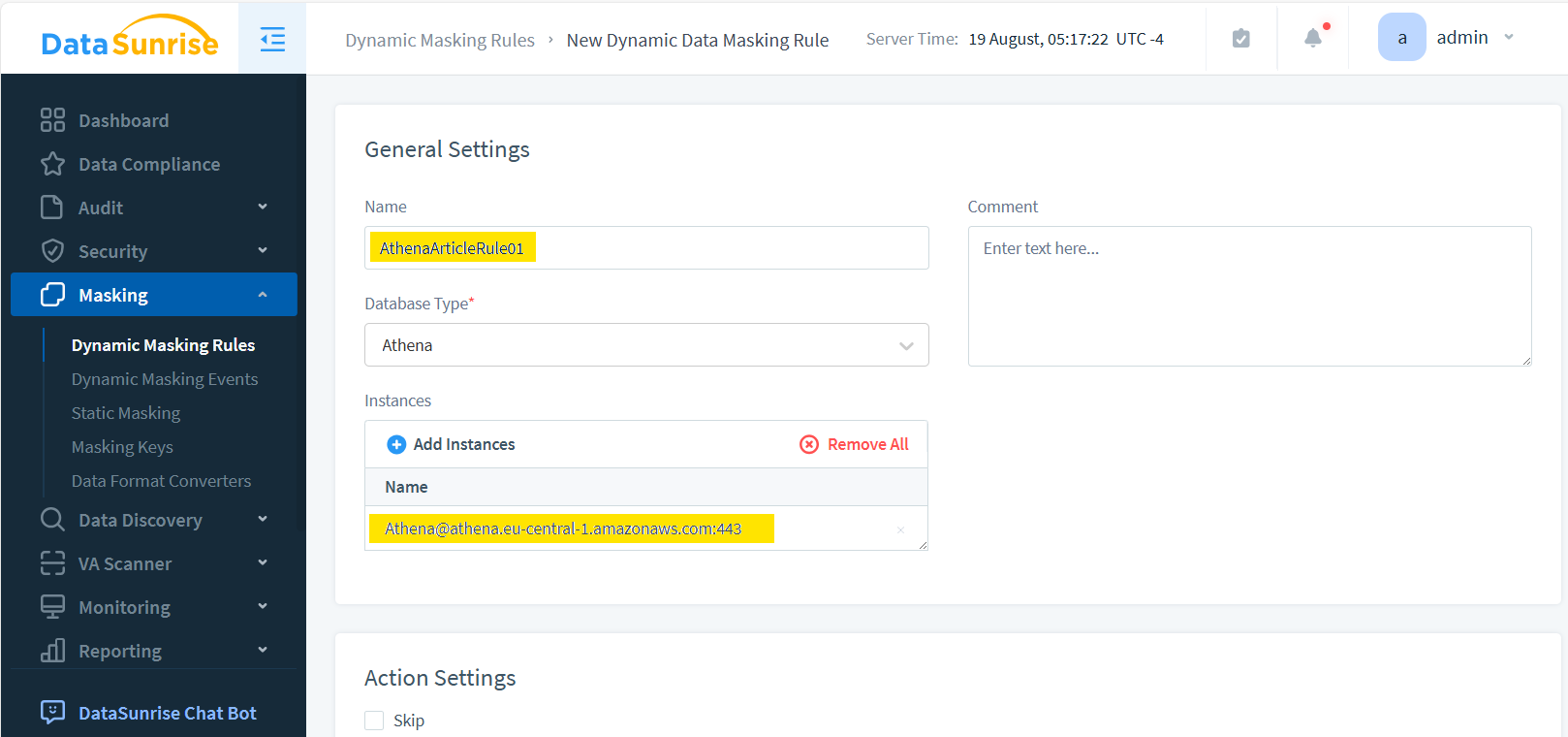
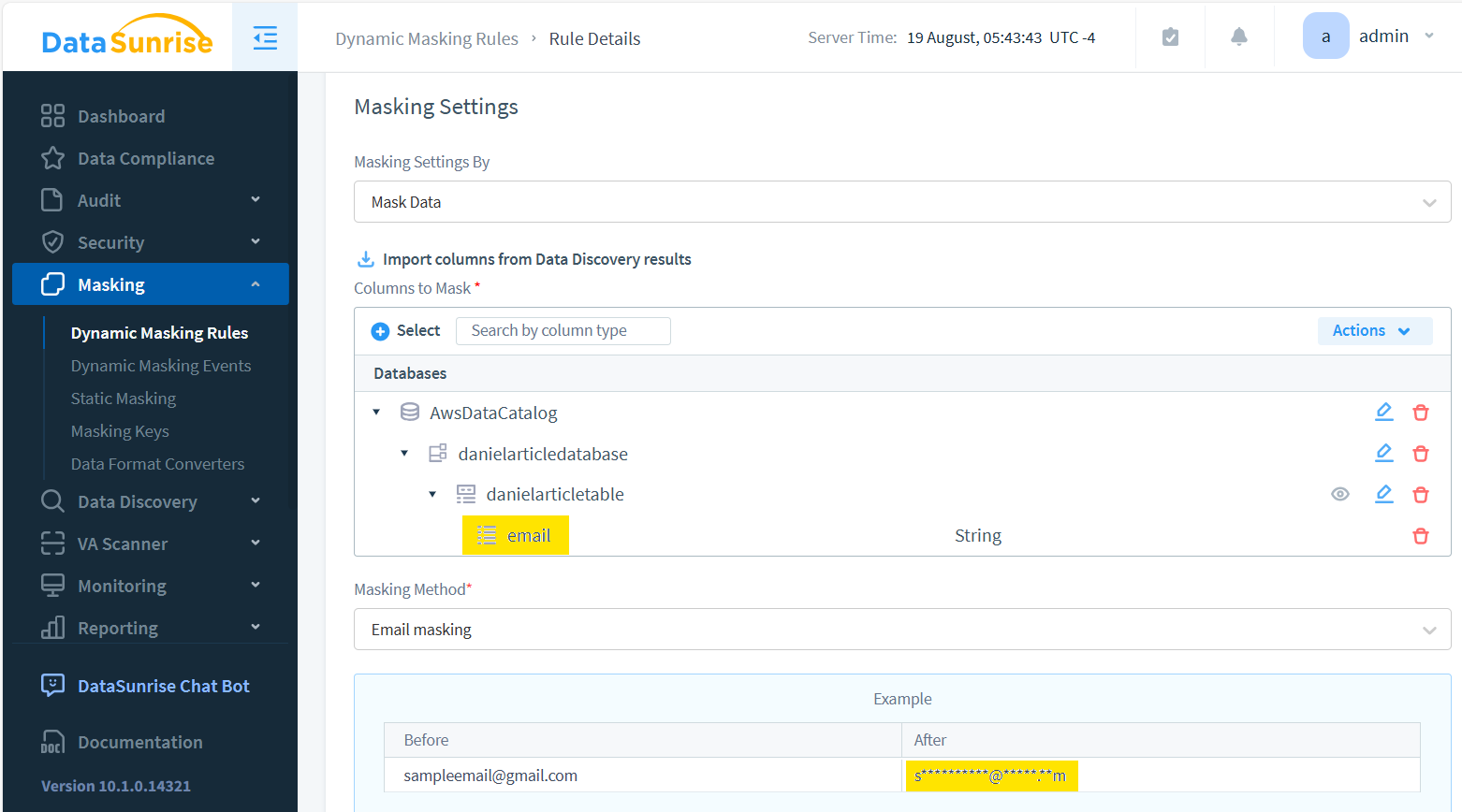
The rule created looks like this:
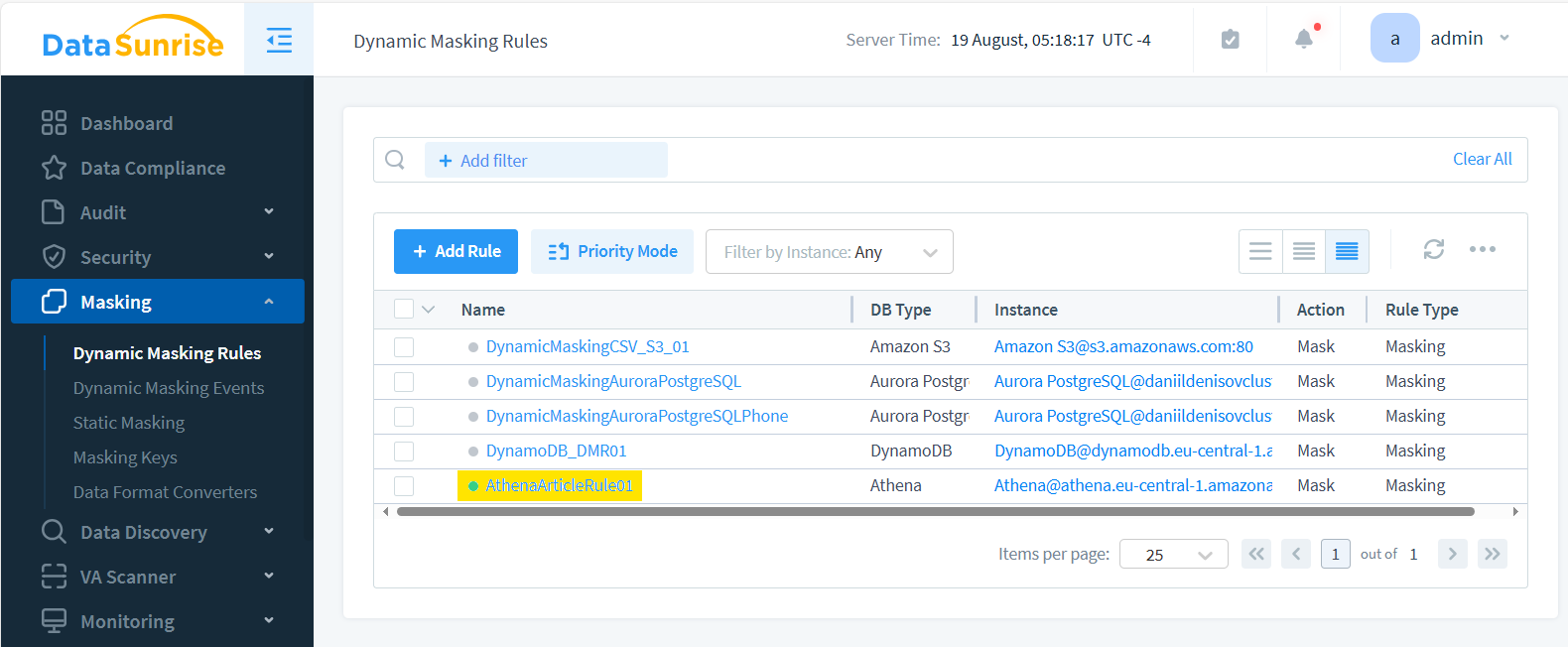
- Query your data through DataSunrise to apply dynamic masking
DataSunrise offers centralized control over masking rules across your entire data setup, ensuring consistent protection.
Accessing DataSunrise Athena Proxy
You should have the following variables set in Python virtual environment (activate.bat script):
set AWS_ACCESS_KEY_ID=your_id_key... set AWS_SECRET_ACCESS_KEY=... set AWS_DEFAULT_REGION=... set AWS_CA_BUNDLE=C:/<YourPath>/certificate-key.txt
To access Athena through the DataSunrise Proxy, follow these steps:
- Navigate to the Configuration – SSL Key Groups page in DataSunrise.
- Select the appropriate instance for which you need the certificate.
- Download the certificate-key.txt file for that instance and save it in the directory specified in AWS_CA_BUNDLE variable.
Once you have the certificate, you can use the following code to connect to Athena via the DataSunrise Proxy at 192.168.10.230:
import boto3 import time import pandas as pd import botocore.config def wait_for_query_to_complete(athena_client, query_execution_id): max_attempts = 50 sleep_time = 2 for attempt in range(max_attempts): response = athena_client.get_query_execution(QueryExecutionId=query_execution_id) state = response['QueryExecution']['Status']['State'] if state == 'SUCCEEDED': return True elif state in ['FAILED', 'CANCELLED']: print(f"Query failed or was cancelled. Final state: {state}") return False time.sleep(sleep_time) print("Query timed out") return False # Configure the proxy connection_config = botocore.config.Config( proxies={'https': 'http://192.168.10.230:1025'}, ) # Connect to Athena with proxy configuration athena_client = boto3.client('athena', config=connection_config) # Execute query query = "SELECT * FROM danielArticleDatabase.danielArticleTable" response = athena_client.start_query_execution( QueryString=query, ResultConfiguration={'OutputLocation': 's3://danielarticlebucket/AthenaArticleTableResults/'} ) query_execution_id = response['QueryExecutionId'] # Wait for the query to complete if wait_for_query_to_complete(athena_client, query_execution_id): # Get results result_response = athena_client.get_query_results( QueryExecutionId=query_execution_id ) # Extract column names columns = [col['Label'] for col in result_response['ResultSet']['ResultSetMetadata']['ColumnInfo']] # Extract data data = [] for row in result_response['ResultSet']['Rows'][1:]: # Skip header row data.append([field.get('VarCharValue', '') for field in row['Data']]) # Create DataFrame df = pd.DataFrame(data, columns=columns) print("\nDataFrame head:") print(df.head()) else: print("Failed to retrieve query results")
Possible output (for Jupyter Notebook):

Benefits of Using DataSunrise for Dynamic Data Masking
DataSunrise’s security suite provides several advantages for Athena users:
- Centralized management of masking rules
- Uniform control across multiple data sources
- Advanced masking techniques beyond native Athena capabilities
- Real-time monitoring and alerting
- Compliance reporting tools
These features make DataSunrise a powerful ally in protecting sensitive data in Amazon Athena.
Conclusion
Dynamic data masking for Amazon Athena is a crucial tool in today’s data security landscape. From native SQL features to advanced solutions like DataSunrise, there are multiple ways to implement this protection.
By masking sensitive data, you can:
- Enhance data security
- Simplify compliance efforts
- Maintain data utility while protecting privacy
As data breaches continue to pose significant risks, implementing robust masking strategies is more important than ever.
Remember, the key to effective data protection lies in choosing the right tools and strategies for your specific needs. Whether you opt for native Athena features or more comprehensive solutions, prioritizing data masking is a step towards a more secure data environment.
DataSunrise offers a comprehensive suite of database security tools, including audit and compliance features. These user-friendly solutions provide flexible and powerful protection for your sensitive data. To see these tools in action and explore how they can enhance your data security strategy, visit our website to schedule an online demo.