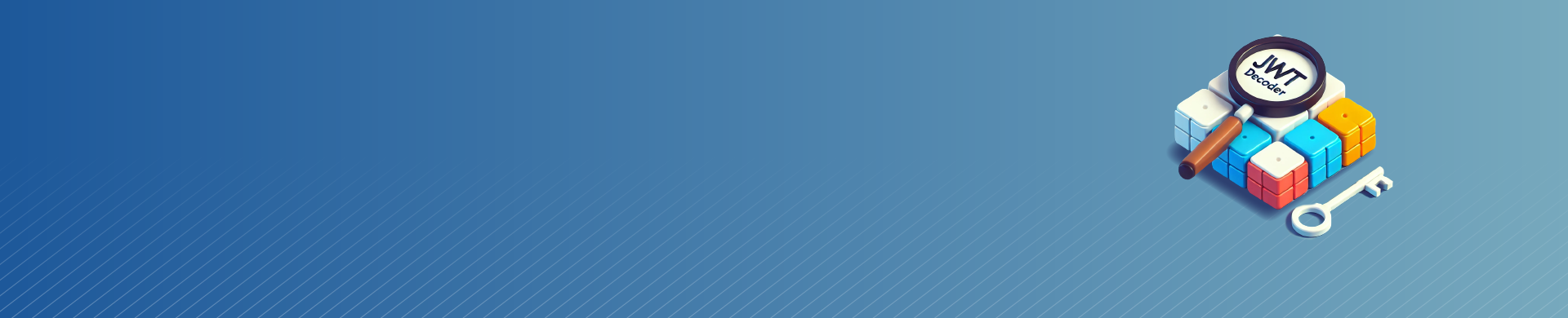
JWT Decoders
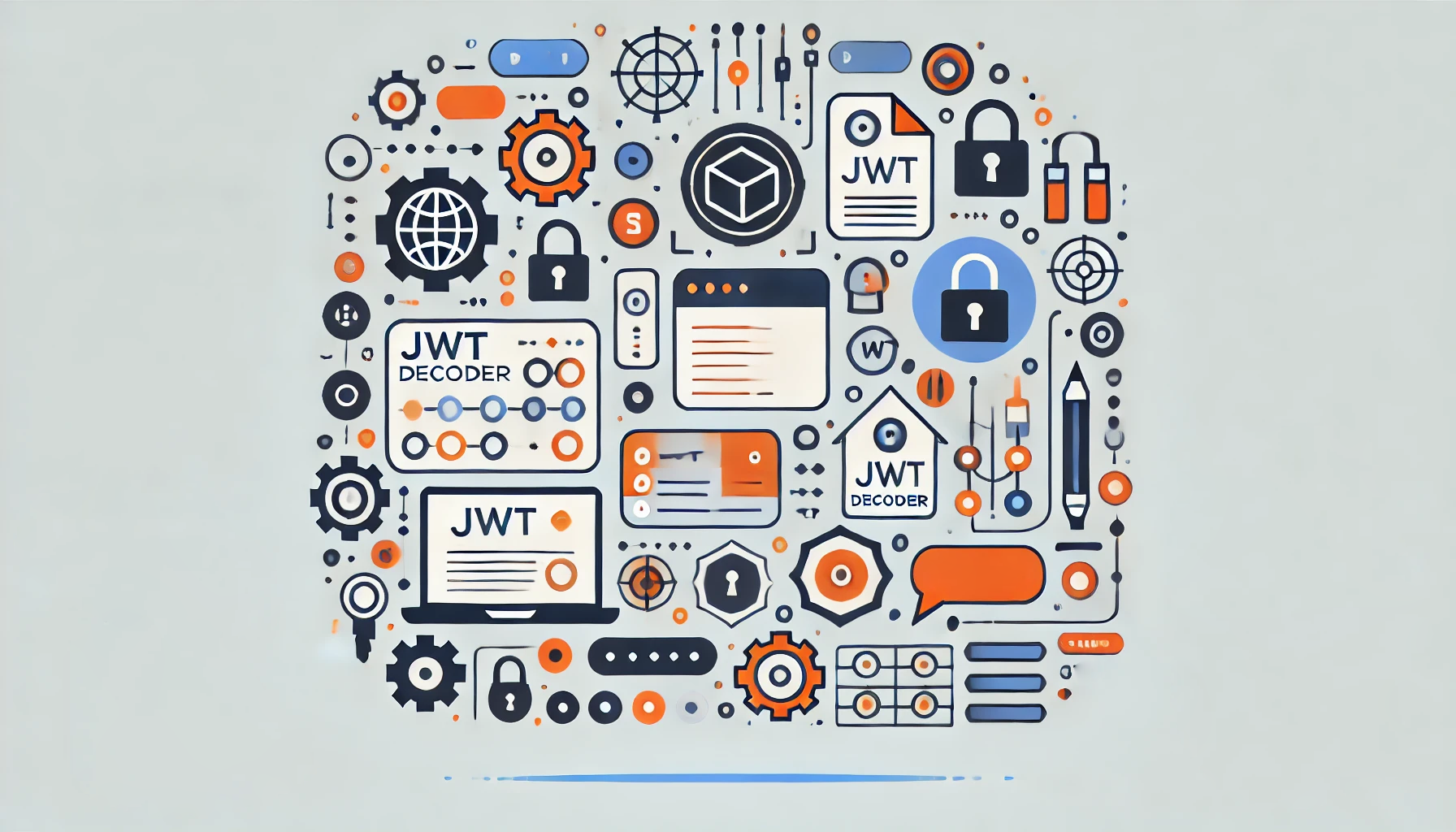
Introduction
In today’s digital landscape, secure authentication and authorization are paramount. JWTs are a secure way to share information between parties in a compact and self-contained manner. However, to harness the full potential of JWTs, we need to understand how to decode and verify them. JWT decoders utilize this role.
This article will dive deep into the world of JWT decoders, exploring their functionality, importance, and practical applications. We will cover everything from the basics of JWTs to advanced decoding techniques. This will help you understand how to create strong authentication systems.
What is a JWT?
Let’s briefly recap what a JWT is and why it’s so widely used before we delve into JWT decoders.
A JSON Web Token (JWT) is a standard way to securely transmit information between parties as a JSON object. Developers commonly use JWTs for authentication and information exchange in web development.
A JWT consists of three parts:
- Header
- Payload
- Signature
These parts are separated by dots and typically look like this:
xxxxx.yyyyy.zzzzz
Encode the header and payload as JSON objects in Base64Url. The system generates the signature by using a secret key and the information from the header and payload.
Understanding JWT Decoders
What is a JWT Decoders?
A JWT decoder is a tool or library that allows you to parse and read the contents of a JWT. It reverses the encoding process, revealing the information stored in the token’s header and payload.
Why Use JWT Decoders?
JWT decoders serve several crucial purposes:
- Verification: They help verify the token’s integrity and authenticity.
- Debugging: Developers use them to inspect token contents during development.
- User management: They allow systems to extract user information from tokens.
- Security auditing: They assist in checking for potential vulnerabilities in token usage.
How JWT Decoders Work
JWT decoders perform several steps to extract and verify the information within a token:
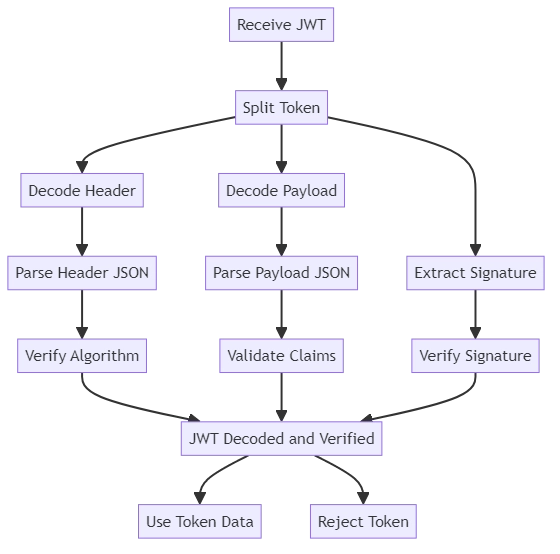
- Splitting the token: The decoder separates the three parts of the JWT.
- Decoding: It Base64Url decodes the header and payload.
- Parsing: The decoded JSON is parsed into a usable format.
- Signature verification: Optionally, the decoder can verify the token’s signature.
Let’s look at an example of how a basic JWT decoder might work:
import base64 import json def decode_jwt(token): # Split the token into its three parts header, payload, signature = token.split('.') # Decode the header and payload decoded_header = base64.urlsafe_b64decode(header + '==').decode('utf-8') decoded_payload = base64.urlsafe_b64decode(payload + '==').decode('utf-8') # Parse the JSON header_data = json.loads(decoded_header) payload_data = json.loads(decoded_payload) return { 'header': header_data, 'payload': payload_data, 'signature': signature } # Example usage jwt = "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ.SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c" decoded = decode_jwt(jwt) print(json.dumps(decoded, indent=2))
This simple decoder splits the token, decodes the header and payload, and returns the parsed data. In a real-world scenario, you’d also want to verify the signature, which we’ll cover later.
Advanced JWT Decoding Techniques
While basic decoding is straightforward, advanced JWT decoding involves several important considerations:
Token Validation
A crucial aspect of JWT decoding is validating the token. This involves:
- Checking the expiration time (exp claim)
- Verifying the issuer (iss claim)
- Confirming the audience (aud claim)
- Validating any custom claims
Here’s an example of how you might validate some of these claims:
import time def validate_jwt_claims(payload): current_time = int(time.time()) if 'exp' in payload and current_time > payload['exp']: raise ValueError("Token has expired") if 'iss' in payload and payload['iss'] != 'https://yourtrustedissuer.com': raise ValueError("Invalid token issuer") if 'aud' in payload and 'your-app-id' not in payload['aud']: raise ValueError("Invalid token audience") # Use this function after decoding the JWT validate_jwt_claims(decoded['payload'])
Signature Verification
Verifying the signature is crucial for ensuring the token hasn’t been tampered with. This process involves:
- Recreating the signature using the header, payload, and secret key
- Comparing the recreated signature with the one in the token
Here’s a basic example of signature verification:
import hmac import hashlib def verify_signature(token, secret): header, payload, signature = token.split('.') message = f"{header}.{payload}" # Create a new signature new_signature = base64.urlsafe_b64encode( hmac.new(secret.encode(), message.encode(), hashlib.sha256).digest() ).decode('utf-8').rstrip('=') return hmac.compare_digest(new_signature, signature) # Use this function to verify the token's signature is_valid = verify_signature(jwt, "your-secret-key") print(f"Signature is valid: {is_valid}")
Implementing JWT Decoders
Now we know how to decode JWT. Next, we will learn how to create a more advanced JWT decoder:
import base64 import json import hmac import hashlib import time class JWTDecoder: def __init__(self, secret): self.secret = secret def decode(self, token): try: header, payload, signature = token.split('.') decoded_header = self._decode_base64(header) decoded_payload = self._decode_base64(payload) if not self._verify_signature(f"{header}.{payload}", signature): raise ValueError("Invalid signature") self._validate_claims(json.loads(decoded_payload)) return { 'header': json.loads(decoded_header), 'payload': json.loads(decoded_payload) } except Exception as e: raise ValueError(f"Invalid token: {str(e)}") def _decode_base64(self, data): padding = '=' * (4 - (len(data) % 4)) return base64.urlsafe_b64decode(data + padding).decode('utf-8') def _verify_signature(self, message, signature): new_signature = base64.urlsafe_b64encode( hmac.new(self.secret.encode(), message.encode(), hashlib.sha256).digest() ).decode('utf-8').rstrip('=') return hmac.compare_digest(new_signature, signature) def _validate_claims(self, payload): current_time = int(time.time()) if 'exp' in payload and current_time > payload['exp']: raise ValueError("Token has expired") if 'iss' in payload and payload['iss'] != 'https://yourtrustedissuer.com': raise ValueError("Invalid token issuer") if 'aud' in payload and 'your-app-id' not in payload['aud']: raise ValueError("Invalid token audience") # Example usage decoder = JWTDecoder("your-secret-key") jwt = "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ.SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c" try: decoded_token = decoder.decode(jwt) print(json.dumps(decoded_token, indent=2)) except ValueError as e: print(f"Error: {str(e)}")
This implementation includes signature verification and claim validation, providing a more secure and comprehensive JWT decoding solution.
Best Practices for Using JWT Decoders
When working with JWT decoders, consider these best practices:
- Always verify the signature before trusting the token’s contents.
- Validate all relevant claims, especially expiration times.
- Use strong, secret keys for signature verification.
- Implement proper error handling to manage invalid tokens gracefully.
- Regularly update your JWT library to patch any security vulnerabilities.
- Do not store sensitive information in the JWT payload because attackers can easily decode it.
Common Pitfalls and How to Avoid Them
While JWT decoders are powerful tools, there are some common mistakes to avoid:
- Trusting unverified tokens: Always verify the signature before using the token’s information.
- Ignoring expiration times: Consistently check the ‘exp’ claim to ensure the token is still valid.
- Using weak secret keys: Use strong, randomly generated keys for signing and verifying tokens.
- Storing sensitive data in JWTs: Remember that the payload can be easily decoded, so avoid storing sensitive information.
- Not handling decoding errors: Implement proper error handling to manage invalid or tampered tokens.
Conclusion
JWT decoders are essential tools in modern web development, enabling secure authentication and authorization systems. By understanding how they work and implementing them correctly, you can enhance the security and functionality of your applications.
Remember, while JWTs offer many benefits, they’re not a silver bullet for all authentication scenarios. Always consider your specific security requirements and choose the most appropriate solution for your needs.
As you continue to work with JWTs and decoders, stay informed about best practices and potential vulnerabilities. The landscape of internet security is constantly evolving. Remaining updated is crucial to maintaining robust and secure systems.