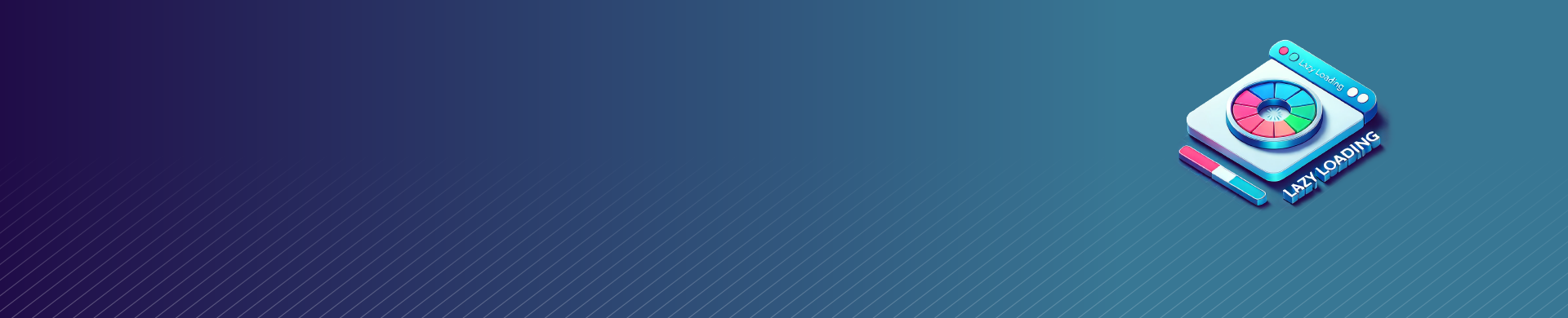
Lazy Loading Explained: Optimizing Resource Loading
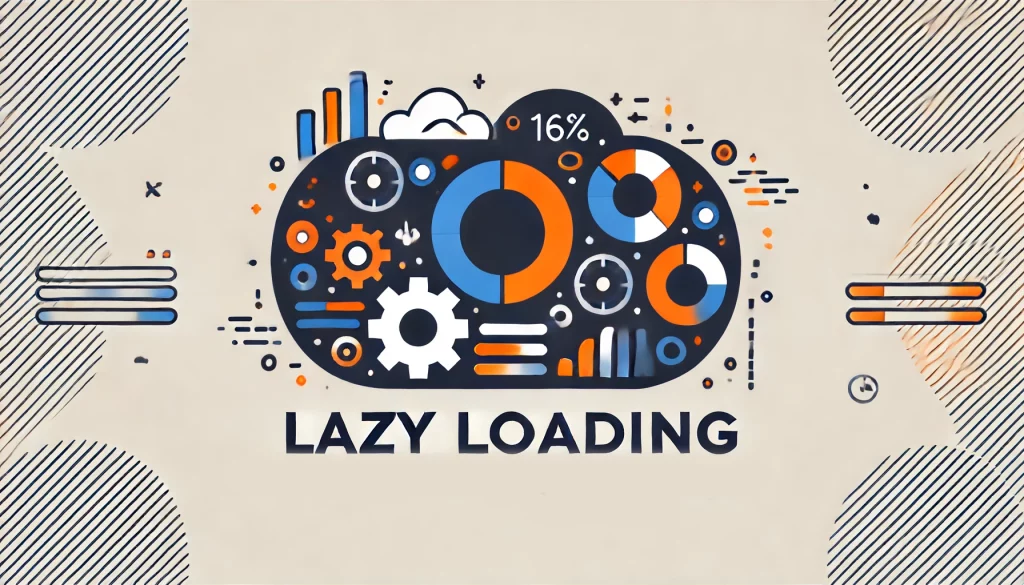
Introduction
Lazy loading is a design pattern and optimization technique where the system loads resources only when needed. Instead of loading everything when the page first loads, lazy loading improves page load times and performance. This is particularly useful for pages with a lot of content below the fold that users might not scroll down to see.
Eager loading fetches and loads all resources immediately when the page loads, regardless of immediate need. This can slow down the initial page load. Lazy loading speeds up the page by loading non-critical resources only when the browser needs them.
Developers often use lazy loading for images. It can also apply to JavaScript files, CSS, or any non-essential content for the initial page view. This method conserves network and computing resources, ensuring a faster user experience by loading these resources only when needed.
Benefits of Lazy Loading
The main advantages of implementing lazy are:
- Faster initial load times: Lazy reduces the page’s initial load time by fetching and rendering only the resources immediately visible to the user. This optimization helps improve user experience and performance. This provides a quicker initial render of the page.
- Reduced bandwidth and resource usage: Lazy downloads resources only if and when the user needs them. If a user never scrolls down to a lazy-loaded image, that image is never fetched, saving bandwidth for both the user and the server.
- Improved performance: By reducing the amount of data that needs to be loaded upfront, lazy puts less strain on the user’s network connection, processor, and memory. This leads to better overall performance and responsiveness, especially on slower networks or lower-end devices.
- Better user experience: Faster load times and improved performance translate into a more positive and engaging user experience. Users can start interacting with the page sooner and experience less lag or delays.
However, lazy is not always the best approach for every situation. For resources that are critical to the page or above the fold, eager might be preferable to ensure they are available immediately. Lazy loading may also not be necessary for small or lightweight resources where the overhead of implementing lazy loading outweighs the benefits.
Eager vs. Lazy Loading
When deciding between lazy and eager loading, consider the following:
- Visibility: If a resource is immediately visible to the user above the fold, eager loading might be the better choice to avoid any delay in loading that content. Lazy is better suited for resources that appear below the fold or require some user interaction to be shown.
- Importance: Critical resources that the page depends on should be eager to avoid delays. Secondary or optional resources are good candidates for lazy loading.
- Size: Lazy loading is most beneficial for large or heavy resources that take significant time to load. Smaller resources may not be worth the overhead of lazy.
- User Behavior: Consider how users typically interact with the page. If analytics show that most users scroll down and view below-the-fold content, eager some of that content might provide a better experience. If few users scroll, lazy loading will minimize unnecessary downloads.
- Network Speed: On slower networks, lazy loading provides bigger performance benefits by reducing the upfront download size. On faster networks, eager may be less detrimental to the user experience.
The right balance of eager and lazy loading will depend on the specific characteristics and requirements of each page or application. Performance testing and monitoring real user metrics can help optimize the approach.
Lazy Loading Implementation Methods
There are several ways to implement lazy loading in web pages:
- JavaScript: Using JavaScript, you can detect when an element enters the viewport and dynamically load its associated resource. Libraries like lazysizes or lazyload make this easier. Example:
- Intersection Observer API: The Intersection Observer API provides a native way to detect when an element enters the viewport and take an action like triggering a load. Example:
- HTML Attributes: Modern browsers support lazy images natively using the loading=”lazy” attribute. Example:
- CSS: CSS techniques can be used to create placeholder skeletons that show while content is loading. Example:
- HTTP/2 Server Push: HTTP/2 provides a server push mechanism where the server can preemptively send resources to the client in anticipation of future requests. While not strictly lazy, this can be used to achieve a similar effect.
<img data-src="image.jpg" class="lazyload">
js
lazyLoadInstance = new LazyLoad({ elements_selector: ".lazyload" });
js
let observer = new IntersectionObserver(callback, options); observer.observe(element);
<img src="image.jpg" loading="lazy" alt="...">
css
.lazy-loaded { background: #f6f7f8; background: linear-gradient(to right, #eeeeee 8%, #dddddd 18%, #eeeeee 33%); background-size: 800px 104px; }
The choice of lazy loading method depends on the specific requirements, browser support considerations, and the overall architecture of the application.
Lazy Loading Images
Images are prime candidates for lazy since they often make up a significant portion of a page’s weight. There are a few ways to lazy load images:
- Native loading Attribute: The most straightforward way is using the browser’s native loading attribute. Set loading=”lazy” on the <img> tag:
- JavaScript: Use JavaScript to check if the image is in the viewport and set the src attribute dynamically:
- CSS: Use CSS to create a placeholder effect while the image loads:
<img src="image.jpg" alt="..." loading="lazy">
<img data-src="image.jpg" alt="..."> <script> const images = document.querySelectorAll('img[data-src]'); const loadImage = (image) => { image.setAttribute('src', image.getAttribute('data-src')); image.onload = () => { image.removeAttribute('data-src'); }; }; const callback = (entries, observer) => { entries.forEach(entry => { if (entry.isIntersecting) { loadImage(entry.target); observer.unobserve(entry.target); } }); }; const options = { rootMargin: '0px 0px 500px 0px' }; const observer = new IntersectionObserver(callback, options); images.forEach(image => observer.observe(image)); </script>
html
<div class="lazy-image"> <img data-src="image.jpg" alt="..."> </div>
css
.lazy-image { background: #f6f7f8; background: linear-gradient(to right, #eeeeee 8%, #dddddd 18%, #eeeeee 33%); background-size: 800px 104px; }
Remember to provide appropriate alt text for accessibility and to handle error cases gracefully.
Conclusion
Lazy loading is a valuable technique for improving the performance and user experience of web pages by deferring the loading of non-critical resources until they are needed. It can significantly reduce initial page load times, save bandwidth, and improve overall performance.
When deciding whether to use lazy loading, consider factors like the visibility and importance of the resource, its size, typical user behavior, and network conditions. Strike a balance between lazy and eager based on the specific needs of your pages.
Lazy can be implemented using various methods like JavaScript, the Intersection Observer API, native HTML attributes, CSS techniques, or server-side optimizations like HTTP/2 server push.
Images are commonly lazy-loaded, but the technique can be applied to any type of content or resource to optimize loading and performance.
By reducing data transfer and optimizing resource loading, lazy not only improves performance but can also help mitigate potential data leaks or breaches that may occur due to transferring more data than necessary.