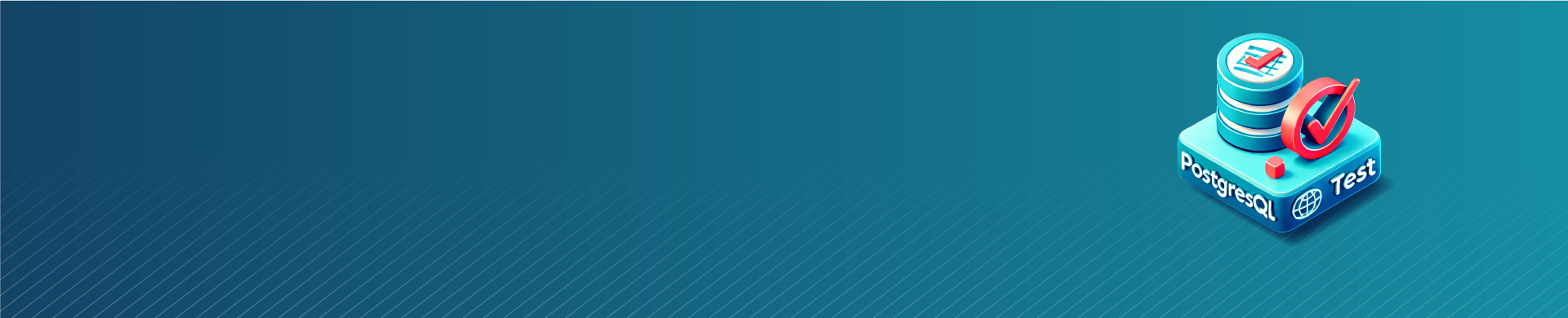
PostgreSQL Test
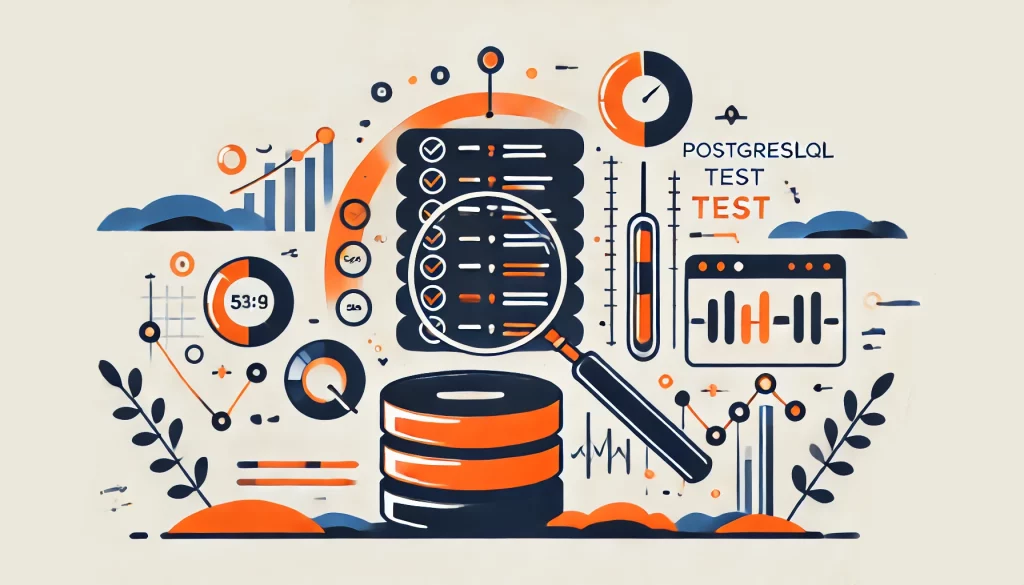
Introduction
Testing is a crucial part of developing robust and reliable applications using PostgreSQL. Testing your PostgreSQL database and code early can help find bugs, keep data accurate, and boost confidence in your software. This article will explore the basics of PostgreSQL test and provide examples to help you get started.
Why Test PostgreSQL Database?
Before diving into the specifics of testing in PostgreSQL, let’s consider why testing is so important:
- Catching bugs early: Testing finds problems in your database, queries, and code before they go live. Catching these problems early can save significant time and effort down the line.
- Ensuring data integrity: Testing your database helps to make sure that your data remains accurate and consistent. This is important because updates may change your application and you may need to update the schema or queries. By testing your database, you can ensure that these changes do not negatively impact the integrity of your data.
- Building confidence: Having a reliable database is important for ensuring that your application functions properly and can handle various situations and special cases.
PostgreSQL Test: Types
There are several types of testing you can perform in PostgreSQL:
1. Unit Testing
Unit testing focuses on testing individual components or functions in isolation. In PostgreSQL, testing SQL queries or functions is important to make sure they give the right results.
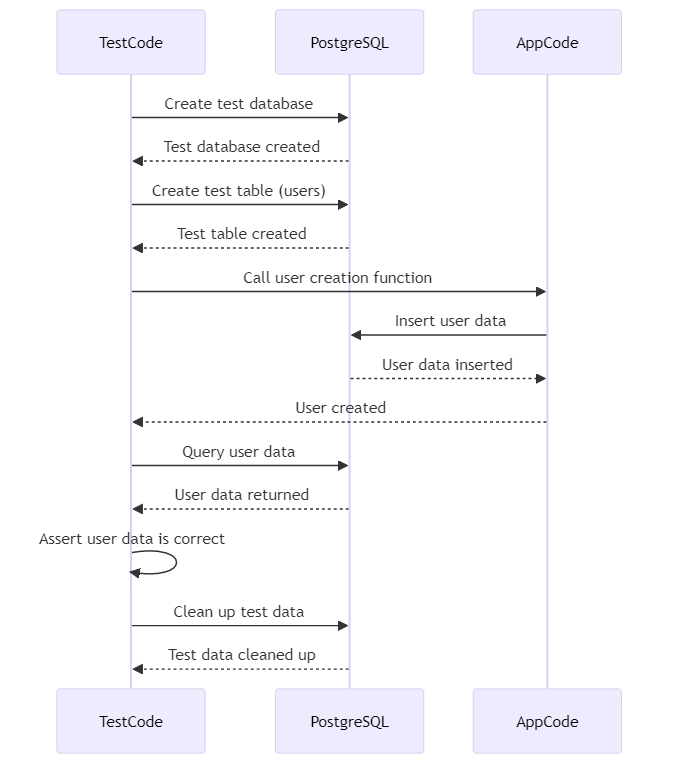
Example:
-- Create a test table CREATE TABLE users ( id SERIAL PRIMARY KEY, name VARCHAR(255) NOT NULL, email VARCHAR(255) UNIQUE NOT NULL ); -- Insert test data INSERT INTO users (name, email) VALUES ('Alice', '[email protected]'), ('Bob', '[email protected]'); -- Test a query SELECT * FROM users WHERE email = '[email protected]';
2. Integration Testing
Integration testing involves testing how different components of your application work together, including the interaction between your application code and the PostgreSQL database. This type of testing ensures that your system’s various parts integrate properly and function as expected.
Example:
import psycopg2 def test_user_creation(): conn = psycopg2.connect("dbname=test_db user=postgres password=secret") cur = conn.cursor() cur.execute("INSERT INTO users (name, email) VALUES (%s, %s)", ("Carol", "[email protected]")) conn.commit() cur.execute("SELECT * FROM users WHERE email = %s", ("[email protected]",)) user = cur.fetchone() assert user[1] == "Carol" assert user[2] == "[email protected]" cur.close() conn.close()
3. Performance Testing
Performance testing shows how well your PostgreSQL database handles heavy use and large amounts of data. This type of testing can help identify bottlenecks and optimize your database for better performance.
Example using pgbench:
pgbench -i -s 50 my_db pgbench -c 10 -j 2 -t 1000 my_db
Setting Up a Test PostgreSQL Environment
To ensure your tests don’t affect your production database, it’s essential to set up a separate test environment. Here’s an example of how you can create a test database in PostgreSQL:
-- Create a test database CREATE DATABASE test_db; -- Connect to the test database \c test_db; -- Create a test schema CREATE SCHEMA test;
Create a separate test database and schema to run tests safely without affecting your production data.
Writing Effective PostgreSQL Test
When writing tests for your PostgreSQL database, consider the following tips:
- Test for edge cases: Make sure to test not only the happy path but also various edge cases and error conditions to ensure your database behaves as expected in all scenarios.
- Use realistic test data: Use test data that closely resembles your production data to get a more accurate picture of how your database will perform in real-world situations.
- Automate your tests: Automate your PostgreSQL tests using testing frameworks and continuous integration tools to ensure they are run regularly and consistently.
- Test at different levels: Write tests at different levels (unit, integration, performance) to cover various aspects of your database and application.
Conclusion
Testing your PostgreSQL database is a vital part of building robust and reliable applications.
Learn the fundamentals of testing in PostgreSQL, create a good test environment, and use best practices for writing tests. This will help you find bugs early, maintain data accuracy, and feel more confident in your software.
Test at different levels, automate tests, and use realistic data to maximize testing efforts. With a solid testing strategy in place, you can develop applications that are more stable, performant, and maintainable.