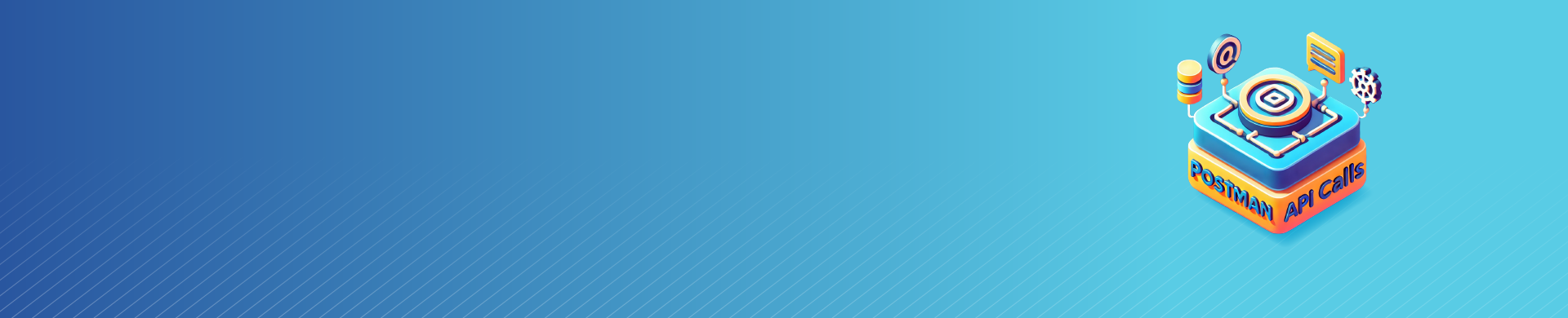
Postman API Calls
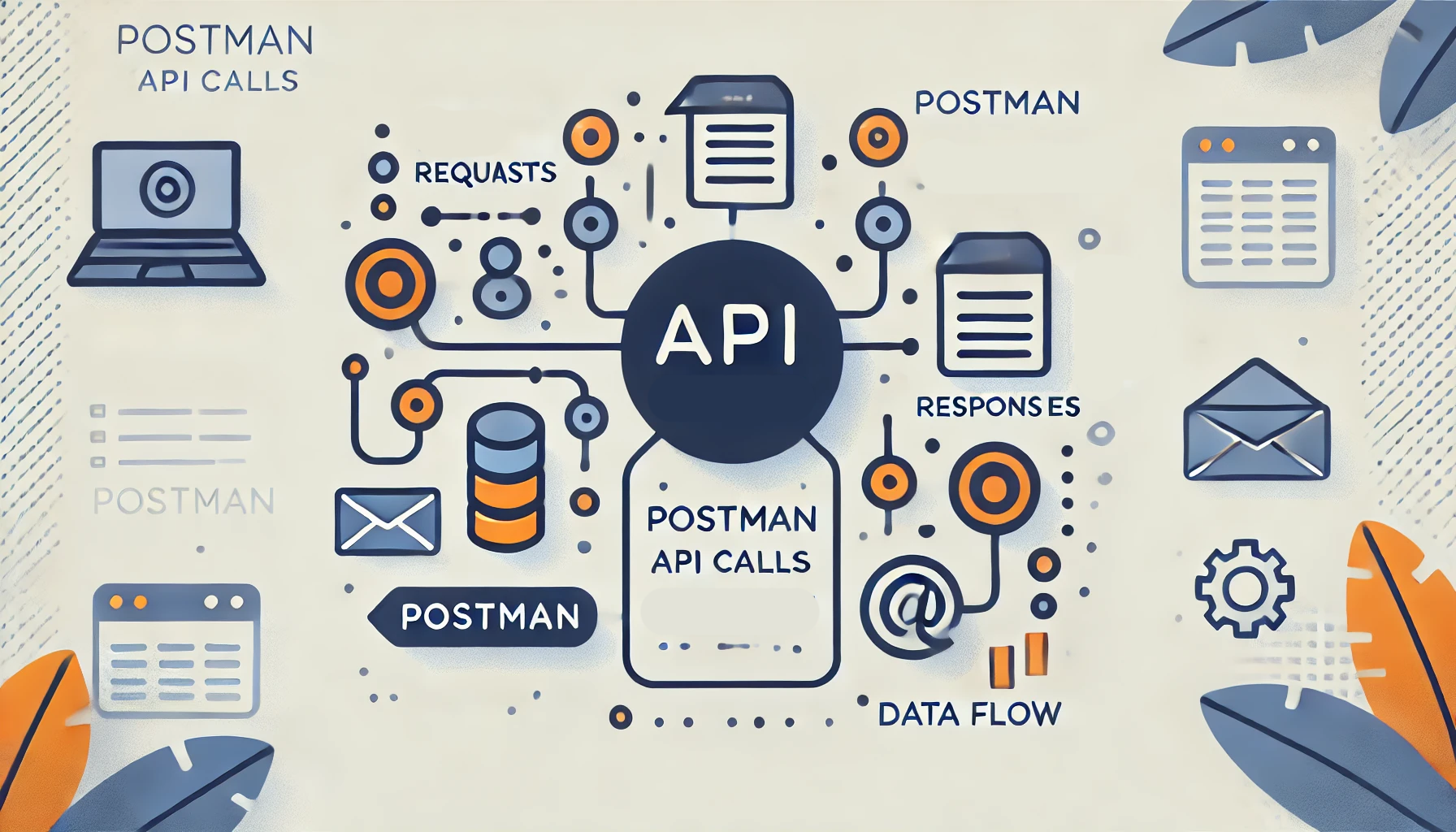
Introduction
This article will dive deep into the fundamentals of web-based services, equipping you with the knowledge and practical examples needed to become proficient in making Postman API calls.
Web-based services have been a crucial component of modern applications for over two decades, evolving significantly since the early 2000s. These services rely heavily on APIs (Application Programming Interfaces) to communicate and exchange data. As developers and testers, we need robust tools to interact with these APIs effectively. This is where Postman comes into play.
DataSunrise Web UI is based on API calls using RPC and JSON-based POST requests. Even when a command is executed in the Command Line Interface (CLI), the required JSON is created based on the command’s options, which is then passed to the backend to call the required RPC. This process is also utilized in the Web Console to create and manage rules and other configurations.
What is Postman?
Postman is a popular API development and testing tool that simplifies the process of sending requests, analyzing responses, and managing APIs. It provides a user-friendly interface for creating and executing API calls, making it easier for developers to work with web services.
Understanding Web-Based Services
Web-based services are applications or components that communicate over networks using standard web protocols. These services enable different software systems to interact and exchange data seamlessly, regardless of their underlying technologies or platforms.
Key Characteristics of Web-Based Services
- Platform Independence: Web services can be accessed from any device or operating system with internet connectivity.
- Interoperability: They allow different systems to communicate using standardized protocols and data formats.
- Scalability: Web services can handle multiple clients and grow as demand increases.
- Reusability: Once created, web services can be used by multiple applications, promoting code reuse and efficiency.
What is RPC?
Remote Procedure Call (RPC) is a protocol (JSON-RPC) that allows a program to execute a procedure or function on another computer as if it were a local procedure call. RPC is one of the earliest forms of distributed computing and has played a significant role in the development of web-based services.
Key Features of RPC:
- Location Transparency: The calling program doesn’t need to know the physical location of the called procedure.
- Synchronous Communication: RPC typically follows a request-response model, where the caller waits for the response before continuing.
- Procedure-Oriented: RPC focuses on calling specific procedures or functions on remote systems.
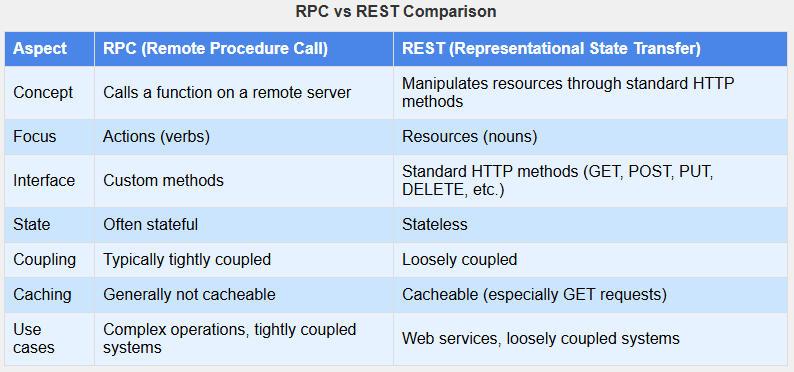
Understanding APIs
An Application Programming Interface (API) is a set of rules, protocols, and tools that allow different software applications to communicate with each other. APIs define the methods and data formats that applications can use to request and exchange information.
Types of APIs
- SOAP (Simple Object Access Protocol): A protocol that uses XML for exchanging structured data.
- REST (Representational State Transfer): An architectural style that uses standard HTTP methods for communication.
- GraphQL: A query language for APIs that allows clients to request specific data they need.
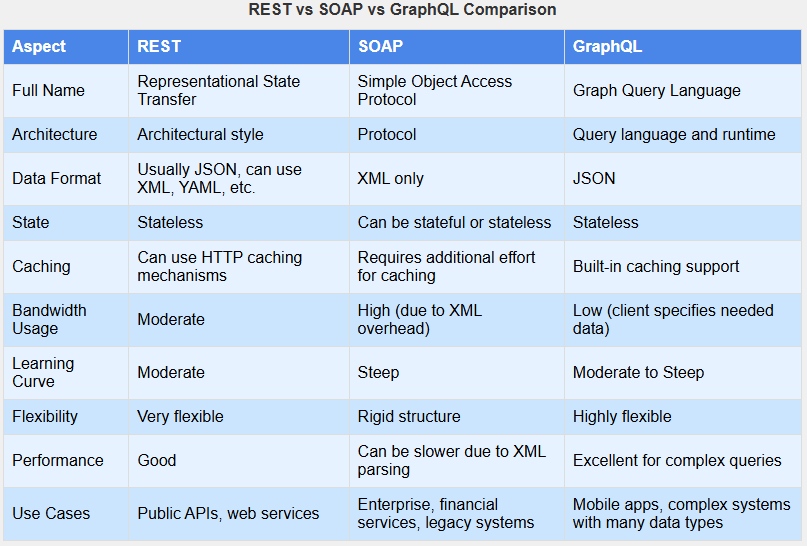
Why APIs are Important
- Integration: APIs enable different systems and applications to work together seamlessly.
- Flexibility: They allow developers to access specific functionalities of other applications without needing to understand their entire codebase.
- Efficiency: APIs can reduce development time by providing pre-built functionalities.
- Scalability: Well-designed APIs can handle increased loads and growing user bases.
Making API Calls with Postman
Now that we understand the basics of web-based services and APIs, let’s explore how to make API calls using Postman. We’ll walk through two examples to demonstrate different types of API calls. https://jsonplaceholder.typicode.com is an API testing website. There are more online.
GET Method: A request to retrieve specific data from a server without modifying any resources, typically used for fetching information and safe for repeated calls.
POST Method: A request to submit data to be processed and stored by the server, often used for creating new resources or submitting form data, which can modify server state.
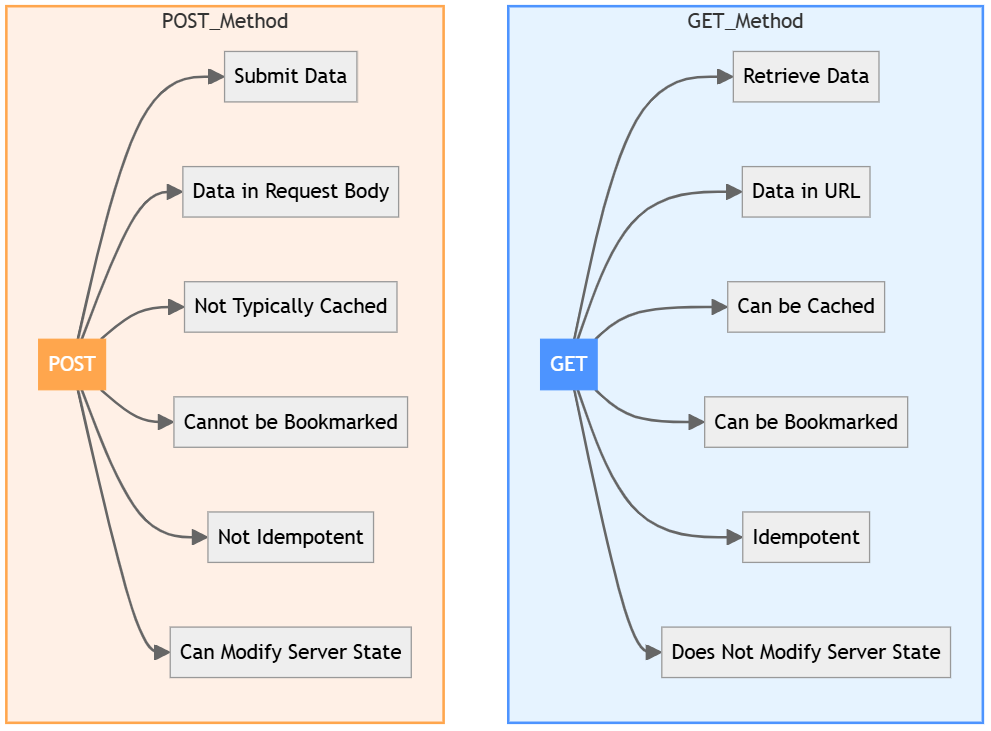
Example 1: Making a GET Request
Let’s start with a simple GET request to retrieve data from a public API.
- Open Postman and create a new request.
- Set the HTTP method to GET.
- Enter the following URL: https://jsonplaceholder.typicode.com/posts/1
- Click the “Send” button.
You should receive a response similar to this:
{ "userId": 1, "id": 1, "title": "sunt aut facere repellat provident occaecati excepturi optio reprehenderit", "body": "quia et suscipit\nsuscipit recusandae consequuntur expedita et cum\nreprehenderit molestiae ut ut quas totam\nnostrum rerum est autem sunt rem eveniet architecto" }
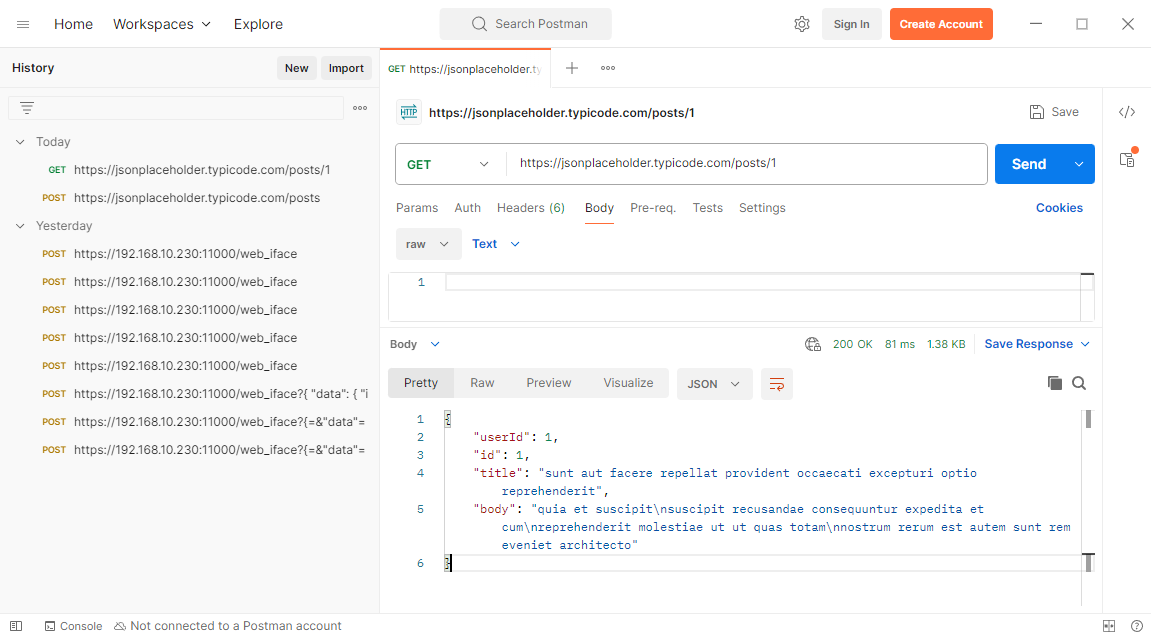
This example demonstrates how easy it is to retrieve data from an API using Postman. The response contains a JSON object with information about a specific post.
Example 2: Making a POST Request
Now, let’s create a new resource using a POST request.
1. Create a new request in Postman.
2. Set the HTTP method to POST.
3. Enter the URL: https://jsonplaceholder.typicode.com/posts
4. Go to the “Body” tab, select “raw,” and choose “JSON” from the dropdown.
5. Enter the following JSON in the body:
jsonCopy{ "title": "My New Post", "body": "This is the content of my new post.", "userId": 1 }
Click the “Send” button.
You should receive a response similar to this:
jsonCopy{ "title": "My New Post", "body": "This is the content of my new post.", "userId": 1, "id": 101 }
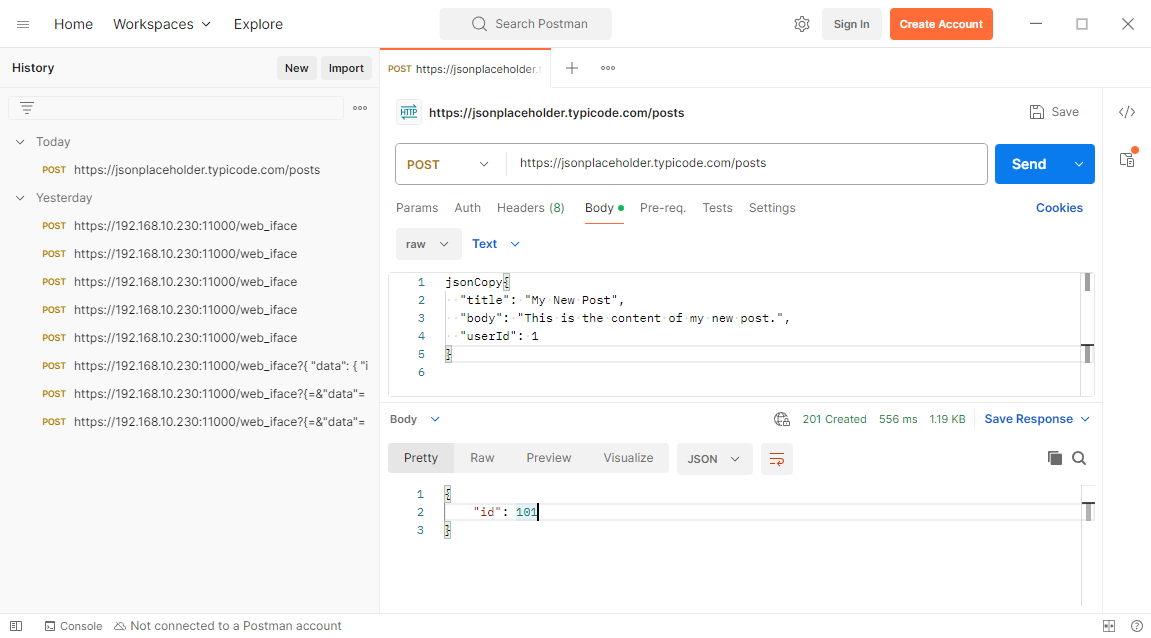
This example shows how to create a new resource using a POST request. The API responds with the created object, including a new id assigned by the server.
If the server uses HTTPS and has a self-signed certificate, you might need to turn off SSL certificate verification in Postman settings.
Advanced Postman Features for API Calls
Postman offers several advanced features that can enhance your API testing and development workflow:
Environment Variables
Postman allows you to create environment variables to store and reuse values across multiple requests. This is particularly useful for managing different API endpoints or authentication tokens.
To use environment variables:
- Create a new environment in Postman.
- Add variables like base_url or api_key.
- Use these variables in your requests with double curly braces, e.g., {{base_url}}/api/endpoint.
Pre-request Scripts
You can write JavaScript code that runs before a request is sent. This is useful for generating dynamic data or setting up authentication.
Example pre-request script for generating a timestamp:
pm.environment.set(“timestamp”, new Date().getTime());
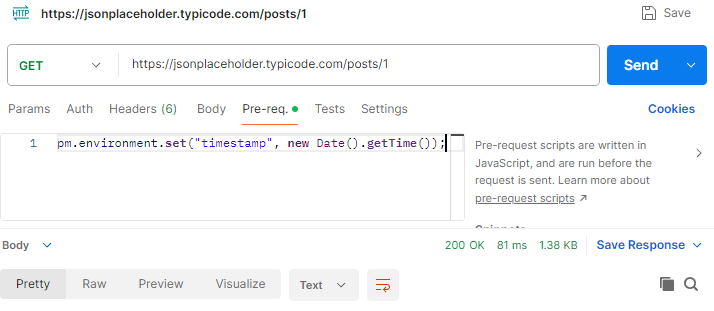
Tests
Postman allows you to write tests for your API calls using JavaScript. These tests can validate response data, check status codes, or perform other assertions.
Example test script in the Tests section of new GET request to https://jsonplaceholder.typicode.com/posts/1?timestamp={{timestamp}}:
// Parse the URL to extract the timestamp var url = pm.request.url.toString(); var timestamp = url.split('timestamp=')[1]; // Log the timestamp console.log("Timestamp used:", timestamp); // Add the timestamp to the response for visibility var responseJson = pm.response.json(); responseJson.timestamp = timestamp; // Set the modified response to visualize in Postman pm.visualizer.set(JSON.stringify(responseJson, null, 2)); // Test to ensure timestamp was sent pm.test("Request sent with timestamp", function () { pm.expect(timestamp).to.not.be.undefined; });
Check the “Test Results” tab to see if the test passed. Also check the “Console” tab to see the logged timestamp. The response body will now include the timestamp value.
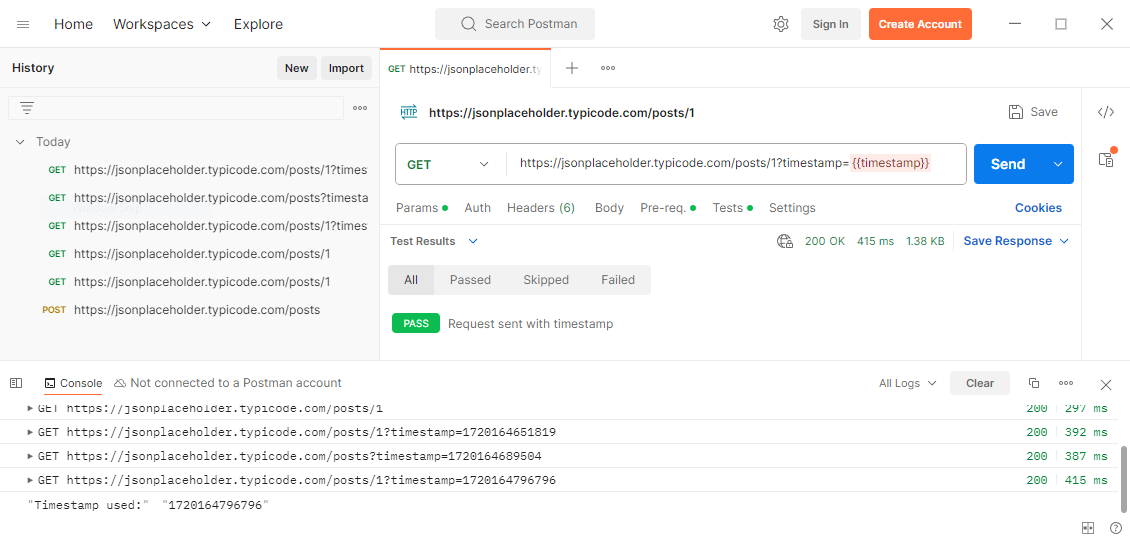
Best Practices for Making API Calls with Postman
- Organize Your Requests: Use collections and folders to group related requests.
- Use Version Control: Postman allows you to export collections. Store these in version control systems like Git.
- Document Your APIs: Use Postman’s documentation feature to create clear, interactive API documentation.
- Automate Testing: Utilize Postman’s Collection Runner and Newman CLI to automate your API tests.
- Handle Authentication Properly: Use environment variables to store sensitive information like API keys or tokens.
- Monitor API Performance: Set up monitors in Postman to track API performance and uptime.
Conclusion
Mastering Postman API calls is an essential skill for modern developers and testers. By understanding web-based services, APIs, and RPC, you can leverage Postman’s powerful features to streamline your API development and testing processes.
We’ve covered the basics of making API calls, explored advanced Postman features, and discussed best practices. With this knowledge, you’re well-equipped to tackle complex API interactions and build robust, interconnected applications.
Remember, the key to becoming proficient with Postman API calls is practice. Experiment with different types of requests, explore public APIs, and challenge yourself to create comprehensive test suites for your own APIs.
As you continue your journey in API development and testing, consider exploring more advanced topics such as API security, performance optimization, and integration testing. The world of web-based services is vast and ever-evolving, offering endless opportunities for learning and growth.